
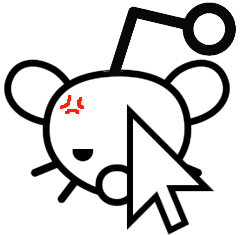
spend less so your employers don’t need to increase your salary. Then they can earn more and get a four levels yacht instead of their crappy three level one.
spend less so your employers don’t need to increase your salary. Then they can earn more and get a four levels yacht instead of their crappy three level one.
I need to eat here…for reasons
Satan 2? What is this high school missile competition?
“I’ve been reborn in the light of the one true God”
“rebellion to tyrants is obedience to gods” lol you mean the being who asks billions to pray to him (sometimes five times a day!) and burns in hell anyone who does not obey?
somehow find it hard to believe they were really offended. it is just another form of satisfying the “being right on the internet” addiction.
what they don’t realize is there is always someone “holier than thou”. you can for instance easily take “comparing Black women to animals” and give it a completely pointless animal rights twist (its not the animal rights that is pointless it is the twist, saying in case someone tries to cancel me) and ask this person to apologise. it is an endless game.
cause everyone knows if conservative replies get upvoted enough people who hate Trump will have a sudden change of heart. Is this guy in the upvote for money business or what?
oh shit it all came flooding back
looks like a scene from the movie gremlins
yea pretty much this, AI is doing some pretty impressive things and even though orders of magnitude faster than a human, no where near in quality as what a human can do as of now (and who knows maybe not even in the next ten years). So any company who treats their employers like “extra costs to be get rid off” deserves the whack of reality.
I don’t know any German but I have been to Germany on multiple occasions and so got this joke!
you and me, we are in the same boat. When I saw these as a kid, I always thought they were to prevent slices from separating and till now had forgotten about them
what about laws preventing social media companies and search engines coming up with algorithms, marketing strategies to maximize time spent in their websites (cough Google cough)
so clearly sun rotates around earth, in your face Galileo - Church probably
lets expand pranks a bit
I am sure if net worth was based strictly on taxed earnings, most rich people would get in line to get their money taxed so that they can boast about it in their yacht owners club meetings
I have heard rumors that it keeps a separate copy of every dinosaur and object for each map and that is why it is so gigantic. hard to guess what would force them such a design though
“one felony count of oral copulation of a person under 18”